Whenever any aggregation function is used, the GROUP BY clause is required. SQL Server 2005 brought the GROUP BY operators like CUBE and ROLLUP, respectively. In addition, SQL Server 2008 brought the GROUP BY operator GROUPING SETS. The GROUPING SETS operator aggregates only specified groups instead of full sets of aggregations by means of CUBE or ROLLUP operators. As an alternative solution for grouping rows in terms of GROUPING SETS operator, one of SET operators was used before SQL Server 2008, the UNION concretely.
USE AdventureWorks2012
GO
SELECT Name,
CountryRegionCode,
SalesLastYear
FROM Sales.SalesTerritory
GO

Figure 1: SSMS result – Basic example skeleton
USE AdventureWorks2012
GO
SET STATISTICS IO ON
GO
SELECT Name,
CountryRegionCode,
SUM(SalesLastYear) AS TotalOfSalesLastYear
FROM Sales.SalesTerritory
GROUP BY Name,
CountryRegionCode
UNION ALL
SELECT Name,
NULL,
SUM(SalesLastYear) AS TotalOfSalesLastYear
FROM Sales.SalesTerritory
GROUP BY Name
UNION ALL
SELECT NULL,
CountryRegionCode,
SUM(SalesLastYear) AS TotalOfSalesLastYear
FROM Sales.SalesTerritory
GROUP BY CountryRegionCode
UNION ALL
SELECT NULL,
NULL,
SUM(SalesLastYear) AS TotalOfSalesLastYear
FROM Sales.SalesTerritory
GO
SET STATISTICS IO OFF
GO

Figure 2: SSMS result – Grouping by means of UNION operator before the GROUPING SETS operator in SQL Server 2008
USE AdventureWorks2012
GO
SET STATISTICS IO ON
GO
SELECT Name,
CountryRegionCode,
SUM(SalesLastYear) AS TotalOfSalesLastYear
FROM Sales.SalesTerritory
GROUP BY
GROUPING SETS
(
(Name, CountryRegionCode),
(Name),
(CountryRegionCode),
()
)
ORDER BY GROUPING(Name),
GROUPING(CountryRegionCode)
GO
SET STATISTICS IO OFF
GO

Figure 3: SSMS result – Grouping by means of GROUPING SETS operator in SQL Server 2008
USE AdventureWorks2012
GO
SET STATISTICS IO ON
GO
SELECT Name,
CountryRegionCode,
SUM(SalesLastYear) AS TotalOfSalesLastYear
FROM Sales.SalesTerritory
GROUP BY
CUBE (Name, CountryRegionCode)
ORDER BY GROUPING(Name),
GROUPING(CountryRegionCode)
GO
SET STATISTICS IO OFF
GO
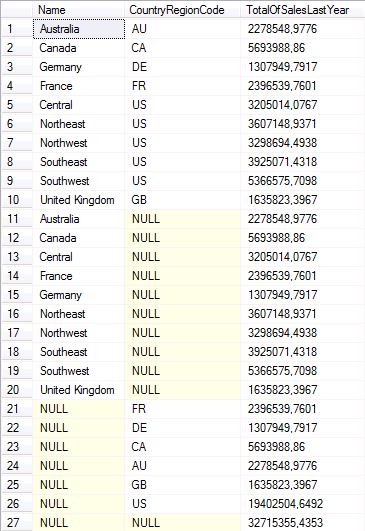
Figure 4: SSMS result – Grouping by means of CUBE operator in SQL Server 2005
The result of IO resources consumption using the UNION ALL operator
by returning 27 rows:
Table 'SalesTerritory'. Scan count 4, logical reads 8,
physical reads 0, read-ahead reads 0,
lob logical reads 0, lob physical reads 0,
lob read-ahead reads 0.
The result of IO resources consumption using the GROUPING SETS operator
by returning 27 rows:
Table 'SalesTerritory'. Scan count 2, logical reads 44,
physical reads 0, read-ahead reads 0,
lob logical reads 0, lob physical reads 0,
lob read-ahead reads 0.
The result of IO resources consumption using the CUBE operator
by returning 27 rows:
Table 'SalesTerritory'. Scan count 2, logical reads 44,
physical reads 0, read-ahead reads 0,
lob logical reads 0, lob physical reads 0,
lob read-ahead reads 0.
- Generating SubTotals using GROUPING
by Sunil Chandurkar - GROUP BY (Transact-SQL)
by MSDN - UNION (Transact-SQL)
by MSDN - Stairway to T-SQL DML Level 8: Using the ROLLUP, CUBE and GROUPING SET operator in a GROUP BY Clause
by Gregory Larsen